bhi160
- Sensor Fusion¶
New in version 1.4.
Supports the BHI160 sensor on the card10 for accelerometer, gyroscope, magnetometer and orientation.
The coordinate system of the BHI160 sensor data looks like this:
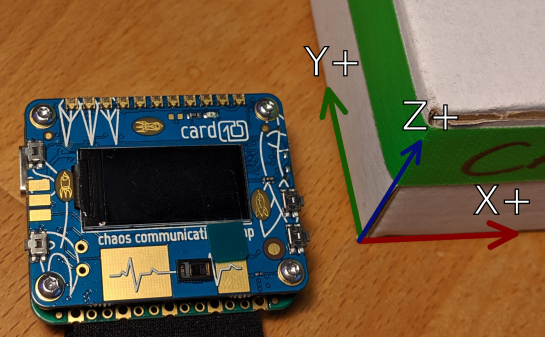
The accelerometer axes are just the ones shown in the picture.
The gyroscope’s angular velocities rotate counter clockwise around their respective axis.
The orientation sensor uses the following mapping:
X
Y
Z
Azimuth (0° - 360°)
Pitch (-180° - 180°)
Roll (-90° - 90°)
Example:
import bhi160
import time
bhi = bhi160.BHI160Orientation()
while True:
samples = bhi.read()
if len(samples) == 0:
time.sleep(0.25)
continue
# print the latest sample
print(samples[-1])
time.sleep(0.25)
Orientation¶
-
class
bhi160.
BHI160Orientation
(sample_rate=4, dynamic_range=2, callback=None, sample_buffer_len=200)[source]¶ Orientation of the BHI160. Orientation is a virtual sensor that combines Accelerometer, Magnetometer and Gyroscope using the IMU Algorithm to calculate an absolute orientation.
This sensors sample data named tuple contains the following fields:
x
: azimuthy
: pitchz
: rollstatus
: accuracy / “confidence” value of the sensor (0 being worst and 3 being best)
Todo
These values are not scaled correctly
- Parameters
sample_rate (int) – Sample rate (optional, default is 4, range is 1 - 200 in Hz)
dynamic_range (int) – This parameter is unused for the Orientation.
callback –
Call this callback when enough data is collected (optional, default is None)
Todo
The callback functionality is untested, so do not be confused if it does not work.
sample_buffer_len (int) – Length of sample buffer (optional, default is 200)
-
close
()¶ Close the connection to the sensor
-
read
()¶ Read sensor values
- Returns
The recent collected sensor values as a list. If no data is available the list contains no elements. Maximum length of the list is
sample_buffer_len
. The last element contains the most recent data. The elements contains a sensor specific named tuple. See the documentation of the sensor class for more information.
Warning
Weird behaviour ahead: If the internal buffer overflows, the new samples will be dropped.
Accelerometer¶
-
class
bhi160.
BHI160Accelerometer
(sample_rate=4, dynamic_range=2, callback=None, sample_buffer_len=200)[source]¶ Accelerometer of the BHI160.
This sensors sample data named tuple contains the following fields:
x
: Acceleration along the x axisy
: Acceleration along the y axisz
: Acceleration along the z axisstatus
: accuracy / “confidence” value of the sensor (0 being worst and 3 being best)
Todo
These values are not scaled correctly
- Parameters
sample_rate (int) – Sample rate (optional, default is 4, range is 1 - 200 in Hz)
dynamic_range (int) – Dynamic range (optional, default is 2)
callback –
Call this callback when enough data is collected (optional, default is None)
Todo
The callback functionality is untested, so do not be confused if it does not work.
sample_buffer_len (int) – Length of sample buffer (optional, default is 200)
-
close
()¶ Close the connection to the sensor
-
read
()¶ Read sensor values
- Returns
The recent collected sensor values as a list. If no data is available the list contains no elements. Maximum length of the list is
sample_buffer_len
. The last element contains the most recent data. The elements contains a sensor specific named tuple. See the documentation of the sensor class for more information.
Warning
Weird behaviour ahead: If the internal buffer overflows, the new samples will be dropped.
Gyroscope¶
-
class
bhi160.
BHI160Gyroscope
(sample_rate=4, dynamic_range=2, callback=None, sample_buffer_len=200)[source]¶ Gyroscope of the BHI160.
This sensors sample data named tuple contains the following fields:
x
: Rotation around the x axisy
: Rotation around the y axisz
: Rotation around the z axisstatus
: accuracy / “confidence” value of the sensor (0 being worst and 3 being best)
Todo
These values are not scaled correctly
- Parameters
sample_rate (int) – Sample rate (optional, default is 4, range is 1 - 200 in Hz)
dynamic_range (int) – Dynamic range (optional, default is 2)
callback –
Call this callback when enough data is collected (optional, default is None)
Todo
The callback functionality is untested, so do not be confused if it does not work.
sample_buffer_len (int) – Length of sample buffer (optional, default is 200)
-
close
()¶ Close the connection to the sensor
-
read
()¶ Read sensor values
- Returns
The recent collected sensor values as a list. If no data is available the list contains no elements. Maximum length of the list is
sample_buffer_len
. The last element contains the most recent data. The elements contains a sensor specific named tuple. See the documentation of the sensor class for more information.
Warning
Weird behaviour ahead: If the internal buffer overflows, the new samples will be dropped.
Magnetometer¶
-
class
bhi160.
BHI160Magnetometer
(sample_rate=4, dynamic_range=1, callback=None, sample_buffer_len=200)[source]¶ Magnetometer of the BHI160
This sensors sample data named tuple contains the following fields:
x
: Magnetic field along the x axisy
: Magnetic field along the y axisz
: Magnetic field along the z axisstatus
: accuracy / “confidence” value of the sensor (0 being worst and 3 being best)
Todo
These values are not scaled correctly
- param int sample_rate
Sample rate (optional, default is 4, range is 1 - 200 in Hz)
- param int dynamic_range
Dynamic range (optional, default is 1)
- param callback
Call this callback when enough data is collected (optional, default is None)
Todo
The callback functionality is untested, so do not be confused if it does not work.
- param int sample_buffer_len
Length of sample buffer (optional, default is 200)
New in version 1.11.
-
close
()¶ Close the connection to the sensor
-
read
()¶ Read sensor values
- Returns
The recent collected sensor values as a list. If no data is available the list contains no elements. Maximum length of the list is
sample_buffer_len
. The last element contains the most recent data. The elements contains a sensor specific named tuple. See the documentation of the sensor class for more information.
Warning
Weird behaviour ahead: If the internal buffer overflows, the new samples will be dropped.